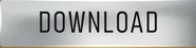

We pointed it to the node ‘p’ and hence added a node to our linked list. The ‘next’ of this prev is holding NULL till now. Prev->next=p – We have used the prev to store the record of the previous node to the current node (the last node from the previous iteration) (you will see in the next line). Head=p – We have given the value to the ‘head’ and thus made the first node of our linked list.Įlse – The linked list is already there and we just have to add a node in this linked list. If(head=NULL) – If the ‘head’ is NULL, then our linked list is not created. We are storing n number of elements in our linked list. This pointer holds the address of the next node and creates the link between two nodes. Each structure represents a node having some data and also a pointer to another structure of the same kind. In C, we achieve this functionality by using structures and pointers. Notice that the last node doesn’t point to any other node and just stores NULL. Here, each node contains a data member (the upper part of the picture) and link to another node(lower part of the picture). It is similar to the picture given below. Every node is mainly divided into two parts, one part holds the data and the other part is connected to a different node. You can also practice a good number of questions from practice section.Ī linked list is made up of many nodes which are connected in nature.
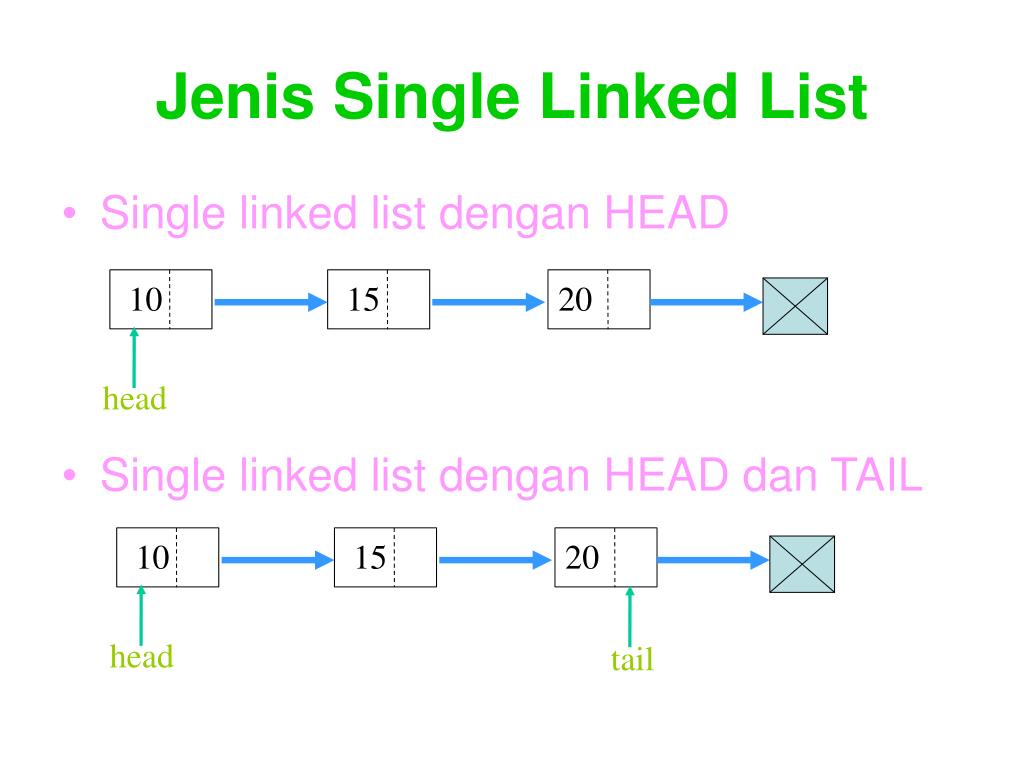
You can go through the pointers chapter if you don’t have a strong grip over it. The implementation of a linked list in C is done using pointers. Linked lists are very useful in this type of situations. We often face situations, where the data is dynamic in nature and number of data can’t be predicted or the number of data keeps changing during program execution. while n.Next 6= fi and n.Next.Linked list is one of the most important data structures.Post: value is removed from the list, true otherwise false.

value is the value to remove from the list.the item to remove doesn't exist in the linked list.the node to remove is somewhere in between the head and tail or.the node to remove is the only node in the linked list or.Post: the item is either in the linked list, true otherwise falseĭeleting a node from a linked list is straight-forward, but there are some cases that you need to account for:.Pre: the head is the head node in the list.Searching a linked list is straightforward: we simply traverse the list checking the value we are looking for with the value of each node in the linked list. Post: value has been placed at the tail of the list.Pre: value is the value to be added to the list.we simply need to append our node onto the end of the list updating the tail reference appropriatelyĪlgorithm for inserting values to Linked List.head = fi, in which case the node we are adding is now both the head and tail of the list or.In general, when people talk about insertion concerning linked lists of any form, they implicitly refer to the adding of a node to the tail of the list.Īdding a node to a singly linked list has only two cases: Here each node makes up a singly linked list and consists of a value and a reference to the next node (if any) in the list. Singly linked lists are one of the most primitive data structures you will learn in this tutorial. the list is dynamic and hence can be resized based on the requirement.Linked lists have a few key points that usually make them very efficient for implementing. As such, linked lists in data structure have some characteristics which are mentioned below: Randomly inserting of values is excluded using this concept and will follow a linear operation. In the data structure, you will be implementing the linked lists which always maintain head and tail pointers for inserting values at either the head or tail of the list is a constant time operation.
#Linked lists series
Linked lists can be measured as a form of high-level standpoint as being a series of nodes where each node has at least one single pointer to the next connected node, and in the case of the last node, a null pointer is used for representing that there will be no further nodes in the linked list.
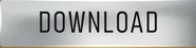